CI4 provides a lot of inbuilt functionalities that simplify handling the data between application and MySQL Database.
Let us look at an example here. We will create a simple form to receive input. File is created under app/Views/users/insert.php
<form name="user" id="insert" method="post" action="save_user">
First Name : <input type="text" name="firstname" /><br/>
Last Name : <input type="text" name="lastname" /><br/>
Age : <input type="text" name="age" /><br/>
Address : <input type="text" name="address" /><br/>
<input type="submit" value="Save" />
</form>
The backend table will be as below: We have set the form textbox name same as the corresponding column name here.
CREATE TABLE `Persons` (
`id` int NOT NULL AUTO_INCREMENT,
`firstname` varchar(50) DEFAULT NULL,
`lastname` varchar(50) DEFAULT NULL,
`age` int DEFAULT NULL,
`address` varchar(255) DEFAULT NULL,
`pincode` int DEFAULT NULL,
`created_date` datetime DEFAULT NULL,
`updated_date` datetime DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4
Now we will set the model file under app/Models/UserModel.php
<?php
namespace App\Models;
use CodeIgniter\Model;
class UserModel extends Model{
protected $table = 'Persons';
protected $primaryKey = 'id';
protected $useAutoIncrement = true; //whether the mentioned primaryKey is an autoincrement field
protected $dateFormat = 'datetime';
protected $createdField = 'created_date';
protected $updatedField = 'updated_date';
protected $useTimestamps = true;
protected $allowedFields = ["firstname","lastname","age","address","pincode"];
}
?>
In the model file, we have set the variables that are inbuilt for CI4.
1. $table : table name for the model.
2. $primaryKey : primary key column name.
3. $useAutoIncrement : whether the mentioned primaryKey is an autoincrement field or not.
4. $dateFormat : we have set value “datetime” to store the date format as “Y-m-d H:i:s”.
5. $createdField: This is the column name for created_date field as in MySQL.
6. $updatedField : This is the column name for updated_date field as in MySQL. This is mandatory if using created_date field. as tested on CI4 version 4.4.3
7. $useTimestamps : If this is true, then CI4 automatically updates the values for created_date and updated_date during insert,update MySQL query.
8. $allowedFields : We have to mention the list of columns that are allowed when using the CI4 model for database reading and writing.
Let us create the controller now under app/Controllers/Home.php
<?php
namespace App\Controllers;
use App\Models;
class Home extends BaseController
{
public function index()
{
return view('users/insert');
}
public function save_user(){
$request = \Config\Services::request();
$postdata = $request->getPost();
//set data for database
$userModel = new \App\Models\UserModel();
$id = $userModel->save($postdata);
if($id == 1){
echo "Saved successfully";
}
}
}
We must set the routing as well for receiving the post value of the form. Routing is set under app/Config/Routes.php
$routes->get('/', 'Home::index');
$routes->post('/save_user', 'Home::save_user');
Now we are all set to test this. Go to the localhost:8080 home page and we can see the form.
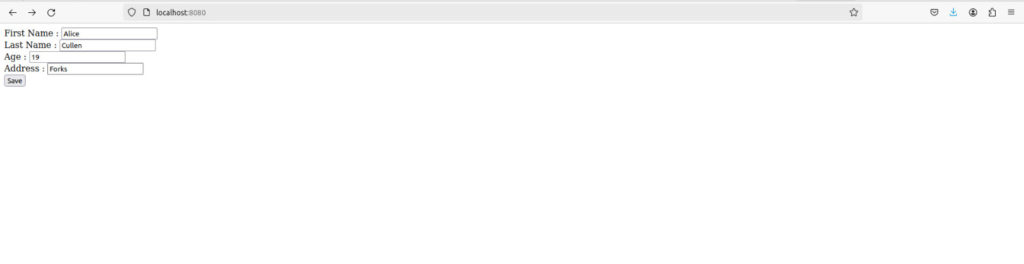
When we click on save we can see that it will be saved in the backend table with the created date and updated date set automatically.
