Codeigniter4 has methods to implement custom pagination design as per our design requirements. We can customise the design and reuse the component across all pages without the need to write the code again and again.
Before we begin, checkout the simple pagination implementation method. We will extend the code from there further to implement the custom design.
First we create the custom pagination design file at app/Views/common/custom_pagination.php
<?php $pager->setSurroundCount(2) ?>
<style>
ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
}
li {
float: left;
}
li a {
display: block;
padding: 16px;
text-align: center;
border-radius: 100%;
color: #ad3d3d;
-webkit-box-shadow: 0 0px 12px 0.8px rgba(0, 0, 0, 0.1);
box-shadow: 0 0px 12px 0.8px rgba(0,0, 0, 0, 0.1);
}
li a:hover {
background-color: #ad3d3d;
color: #fff;
}
</style>
<ul class="pagination">
<?php if ($pager->hasPrevious()) : ?>
<li>
<a href="<?= $pager->getFirst() ?>" aria-label="<?= lang('Pager.first') ?>">
<span aria-hidden="true"><?= lang('Pager.first') ?></span>
</a>
</li>
<li>
<a href="<?= $pager->getPrevious() ?>" aria-label="<?= lang('Pager.previous') ?>">
<span aria-hidden="true"><?= lang('Pager.previous') ?></span>
</a>
</li>
<?php endif ?>
<?php foreach ($pager->links() as $link) : ?>
<li <?= $link['active'] ? 'class="active"' : '' ?>>
<a <?= $link['active'] ? 'style="color: #111;font-weight:bold;"' : '' ?> href="<?= $link['uri'] ?>">
<?= $link['title'] ?>
</a>
</li>
<?php endforeach ?>
<?php if ($pager->hasNext()) : ?>
<li>
<a href="<?= $pager->getNext() ?>" aria-label="<?= lang('Pager.next') ?>">
<span aria-hidden="true"><?= lang('Pager.next') ?></span>
</a>
</li>
<li>
<a href="<?= $pager->getLast() ?>" aria-label="<?= lang('Pager.last') ?>">
<span aria-hidden="true"><?= lang('Pager.last') ?></span>
</a>
</li>
<?php endif ?>
</ul>
Now we have to register this page under app/Config/Pager.php. We will add our pagination template name “custom_pagination_page” and the file path as below.
public array $templates = [
'default_full' => 'CodeIgniter\Pager\Views\default_full',
'default_simple' => 'CodeIgniter\Pager\Views\default_simple',
'default_head' => 'CodeIgniter\Pager\Views\default_head',
'custom_pagination_page' => 'App\Views\common\custom_pagination', //This is our custom page
];
In the controller file as per the previous post, app/Controllers/Home.php we had the pager->makelinks function call. We will add a fourth parameter here, which is the pagination template name as mentioned above.
$data['page_links'] = $pager->makelinks($page,$userModel->limit,$allPersonsCount,'custom_pagination_page');
That’s all. We can see that the pagination with custom design is ready. We can reuse this design across all pages as needed.
Below is a screenshot of the page on localhost.
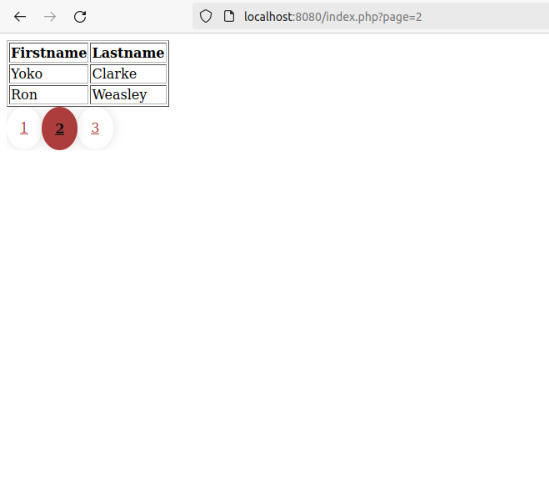