CI4 has a helper for cookies management which contains some functions to implement the cookies. Let us see with an example.
<?php
namespace App\Controllers;
class Home extends BaseController
{
public function index()
{
helper('cookie');
set_cookie("DVXCookie", "SETPCookieTesting", 300, "localhost", '', "SETP_");
echo "Cookie set";
}
}
set_cookie function takes below parameters.
1. Cookie name
2. Cookie value
3. Expiry in seconds (optional)
4. Domain (optional)
5. Cookie Path (optional)
6. Prefix to be added (optional)
You can add a default prefix for all the Cookies in the app/Config/Cookie.php file. The variable $prefix takes the default value which you set as per your requirement. Let’s say we set the $prefix as “MI_”. If we don’t pass any value for prefix in the set_cookie function, CI4 will pick the default prefix value.
When you run the code above, you can see that the cookie is set, which is visible in the developer view on the browser. Below is a screenshot for Chrome browser.
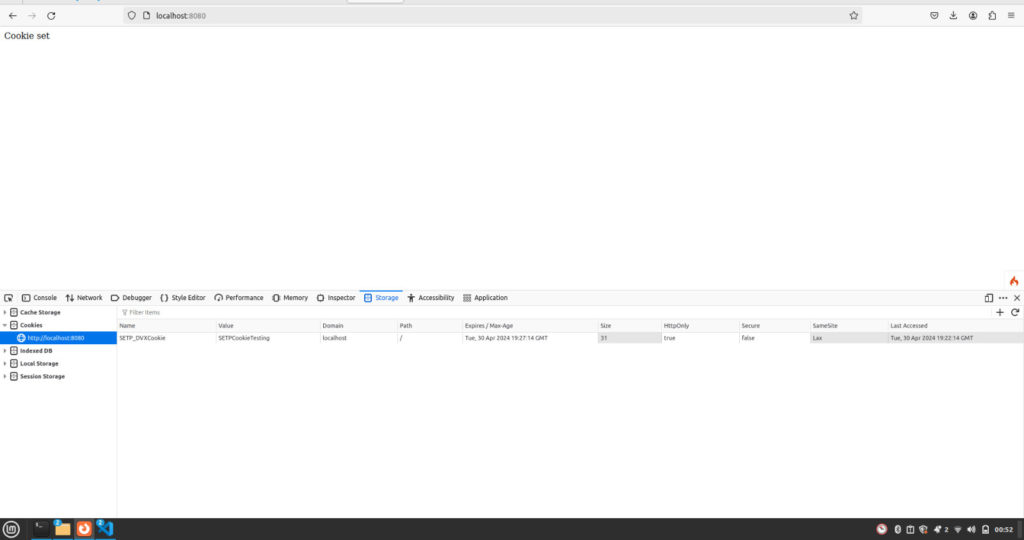
You can also fetch the value of the cookie using a get_cookie function.
get_cookie takes the following parameters.
1. Cookie name
2. boolean value for xss filter
3. prefix value
<?php
namespace App\Controllers;
class Home extends BaseController
{
public function index()
{
helper('cookie');
var_dump(get_cookie("DVXCookie",true,"SETP_"));
}
}
?>
We can see that the set value is returned and printed on the browser. Below is the output.
string(17) “SETPCookieTesting”
We can also delete the cookies from the browser using the delete_cookie function. It takes parameters as below
1. Cookie Name
2. Domain (optional)
3. Path (optional)
4. Prefix (optional)
You can call delete_cookie(“DVXCookie”); and the cookie will be deleted.